jGISTools - Sample 2
Sample 2 - Using the map pane
This sample will show how to use the map pane component. You should have completed the first sample before starting this one.
Overview
The map pane can be considered a simple map viewer that provides out of the box the following tools and actions:
- Select tool
- Drag tool
- Zoom tool (left click for zoom in, right for zoom out)
- Zoom in action (center)
- Zoom out action (center)
- Zoom 1:1
The actions and tools mentioned above are displayed by default by the map pane, however the application developer can specify which actions and tools should be displayed to meet the needs of his application.
Usage
It is extremely easy to use the map pane: just create a map component, create the map pane by specifiying the previously created map component and after that add the map pane to a frame window.
Let's see the code:
/* * 1.Create the specific map model that will * be used by the visual component. We need to supply the CRS to * the map model, so we will use a conventient utiliy method to get that. */ //1.1 Get the crs String path = args[0]; CoordinateReferenceSystem crs = ShapefileMapModel.getCRS(path); //1.2 Check if the CRS could be obtained if( crs == null ) {//no CRS :( throw new RuntimeException("Could not get CRS for the specified shapefile ! Consider building the CRS manually !"); } //1.3 Now create the model object with the obtained crs ShapefileMapModel model = new ShapefileMapModel(crs); //2.Create the map component that will display the map data GUIFactoryBuilder factoryBuilder = GUIFactoryBuilder.getInstance(); IGUIFactory componentFactory = factoryBuilder.getGUIFactory(GuiPlatformType.SWING); IMapComponent mapComponent = componentFactory.createMapComponent(model, null); mapComponent.initializeMapComponent();
After we have created the map component, we create the map pane by invoking:
//3.Create the map pane that will host the map control together with other UI elements IMapPane mapPane = componentFactory.createMapPane(mapComponent, null);
Now that we have the map pane, we can start adding data to the model:
/* 4.Load the map data. This should be called AFTER the map component was set in the map pane, so that the map pane will be able to process the MapLoaded event. */ ShapefileInfo shpInfo = new ShapefileInfo(path); model.addShapefile(shpInfo);//should be called after the model was passed to the map component
And the last step is to add the map pane to a frame window:
//5.Add the map pane from the prev step to a JFrame object and display it JFrame frame = new JFrame("Demo application for IMapPane"); frame.add((JPanel)mapPane.getWidget(), BorderLayout.CENTER); frame.setSize(800, 600); frame.addWindowListener(new WindowEventsHandler()); frame.setVisible(true);
Results
After running the code you should get something like this:
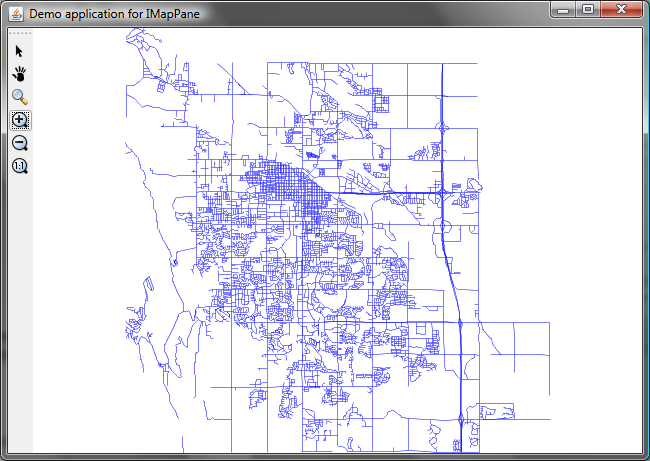
The third sample will present the usage of the library in a real world application, by trying to create a map viewer application.